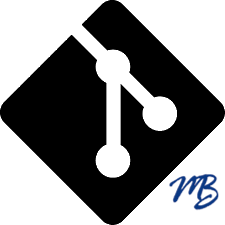
Lots of unit tests for new WAMP classes Fixed parse error in TopicManager Aliased Topic::getId -> __toString to WampConnection can work with Topics or raw protocol strings API docs CS
88 lines
2.3 KiB
PHP
88 lines
2.3 KiB
PHP
<?php
|
|
namespace Ratchet\Tests\Wamp;
|
|
use Ratchet\Wamp\Topic;
|
|
use Ratchet\Wamp\WampConnection;
|
|
|
|
/**
|
|
* @covers Ratchet\Wamp\Topic
|
|
*/
|
|
class TopicTest extends \PHPUnit_Framework_TestCase {
|
|
public function testGetId() {
|
|
$id = uniqid();
|
|
$topic = new Topic($id);
|
|
|
|
$this->assertEquals($id, $topic->getId());
|
|
}
|
|
|
|
public function testAddAndCount() {
|
|
$topic = new Topic('merp');
|
|
|
|
$topic->add($this->newConn());
|
|
$topic->add($this->newConn());
|
|
$topic->add($this->newConn());
|
|
|
|
$this->assertEquals(3, count($topic));
|
|
}
|
|
|
|
public function testRemove() {
|
|
$topic = new Topic('boop');
|
|
$tracked = $this->newConn();
|
|
|
|
$topic->add($this->newConn());
|
|
$topic->add($tracked);
|
|
$topic->add($this->newConn());
|
|
|
|
$topic->remove($tracked);
|
|
|
|
$this->assertEquals(2, count($topic));
|
|
}
|
|
|
|
public function testBroadcast() {
|
|
$msg = 'Hello World!';
|
|
$name = 'Batman';
|
|
$protocol = json_encode(array(8, $name, $msg));
|
|
|
|
$first = $this->getMock('Ratchet\\Wamp\\WampConnection', array('send'), array($this->getMock('\\Ratchet\\ConnectionInterface')));
|
|
$second = $this->getMock('Ratchet\\Wamp\\WampConnection', array('send'), array($this->getMock('\\Ratchet\\ConnectionInterface')));
|
|
|
|
$first->expects($this->once())
|
|
->method('send')
|
|
->with($this->equalTo($protocol));
|
|
|
|
$second->expects($this->once())
|
|
->method('send')
|
|
->with($this->equalTo($protocol));
|
|
|
|
$topic = new Topic($name);
|
|
$topic->add($first);
|
|
$topic->add($second);
|
|
|
|
$topic->broadcast($msg);
|
|
}
|
|
|
|
public function testIterator() {
|
|
$first = $this->newConn();
|
|
$second = $this->newConn();
|
|
$third = $this->newConn();
|
|
|
|
$topic = new Topic('Joker');
|
|
$topic->add($first)->add($second)->add($third);
|
|
|
|
$check = array($first, $second, $third);
|
|
|
|
foreach ($topic as $mock) {
|
|
$this->assertNotSame(false, array_search($mock, $check));
|
|
}
|
|
}
|
|
|
|
public function testToString() {
|
|
$name = 'Bane';
|
|
$topic = new Topic($name);
|
|
|
|
$this->assertEquals($name, (string)$topic);
|
|
}
|
|
|
|
protected function newConn() {
|
|
return new WampConnection($this->getMock('\\Ratchet\\ConnectionInterface'));
|
|
}
|
|
} |