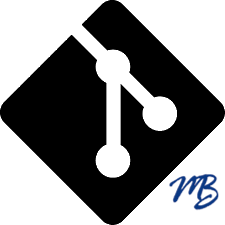
Separated Observable interface from Decorator interface, also separated config method to its own interface Cleaned up unit tests to reflect interface changes
70 lines
2.1 KiB
PHP
70 lines
2.1 KiB
PHP
<?php
|
|
namespace Ratchet\Tests;
|
|
use Ratchet\Tests\Mock\FakeSocket as Socket;
|
|
use Ratchet\Socket as RealSocket;
|
|
use Ratchet\Tests\Mock\Application as TestApp;
|
|
|
|
/**
|
|
* @covers Ratchet\Socket
|
|
*/
|
|
class SocketTest extends \PHPUnit_Framework_TestCase {
|
|
protected $_socket;
|
|
|
|
protected static function getMethod($name) {
|
|
$class = new \ReflectionClass('\\Ratchet\\Tests\\Mock\\FakeSocket');
|
|
$method = $class->getMethod($name);
|
|
$method->setAccessible(true);
|
|
|
|
return $method;
|
|
}
|
|
|
|
public function setUp() {
|
|
$this->_socket = new Socket();
|
|
}
|
|
|
|
public function testGetDefaultConfigForConstruct() {
|
|
$ref_conf = static::getMethod('getConfig');
|
|
$config = $ref_conf->invokeArgs($this->_socket, array());
|
|
|
|
$this->assertEquals(array_values(Socket::$_defaults), $config);
|
|
}
|
|
|
|
public function testInvalidConstructorArguments() {
|
|
$this->setExpectedException('\\Ratchet\\Exception');
|
|
$socket = new RealSocket('invalid', 'param', 'derp');
|
|
}
|
|
|
|
public function testConstructAndCallByOpenAndClose() {
|
|
$socket = new RealSocket();
|
|
$socket->close();
|
|
}
|
|
|
|
public function testInvalidSocketCall() {
|
|
$this->setExpectedException('\\BadMethodCallException');
|
|
$this->_socket->fake_method();
|
|
}
|
|
|
|
public function asArrayProvider() {
|
|
return array(
|
|
array(array('hello' => 'world'), array('hello' => 'world'))
|
|
, array(null, null)
|
|
, array(array('hello' => 'world'), new \ArrayObject(array('hello' => 'world')))
|
|
);
|
|
}
|
|
|
|
/**
|
|
* @dataProvider asArrayProvider
|
|
*/
|
|
public function testMethodMungforselectReturnsExpectedValues($output, $input) {
|
|
$method = static::getMethod('mungForSelect');
|
|
$return = $method->invokeArgs($this->_socket, array($input));
|
|
|
|
$this->assertEquals($return, $output);
|
|
}
|
|
|
|
public function testMethodMungforselectRejectsNonTraversable() {
|
|
$this->setExpectedException('\\InvalidArgumentException');
|
|
$method = static::getMethod('mungForSelect');
|
|
$method->invokeArgs($this->_socket, array('I am upset with PHP ATM'));
|
|
}
|
|
} |