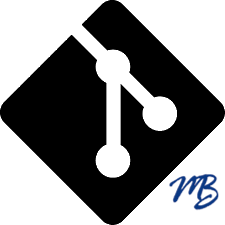
Refactored Command/Composite pattern, now as expected Server recursively executes commands Above changes fixed issues of server/client not being notified on forced disconnects
35 lines
834 B
PHP
35 lines
834 B
PHP
<?php
|
|
namespace Ratchet\Command;
|
|
use Ratchet\SocketObserver;
|
|
|
|
class Composite extends \SplQueue implements CommandInterface {
|
|
public function enqueue(CommandInterface $command) {
|
|
if ($command instanceof Composite) {
|
|
foreach ($command as $cmd) {
|
|
$this->enqueue($cmd);
|
|
}
|
|
|
|
return;
|
|
}
|
|
|
|
parent::enqueue($command);
|
|
}
|
|
|
|
public function execute(SocketObserver $scope = null) {
|
|
$this->setIteratorMode(static::IT_MODE_DELETE);
|
|
|
|
$recursive = new self;
|
|
|
|
foreach ($this as $command) {
|
|
$ret = $command->execute($scope);
|
|
|
|
if ($ret instanceof CommandInterface) {
|
|
$recursive->enqueue($ret);
|
|
}
|
|
}
|
|
|
|
if (count($recursive) > 0) {
|
|
return $recursive;
|
|
}
|
|
}
|
|
} |