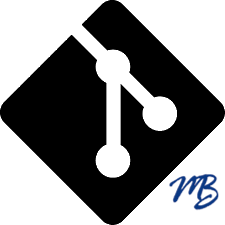
Updated deps; React Socket notify client of shutdown Separated core interfaces into many Removed initial version support out of handshake negotiator Moved message parser responsibility to each version Removed __toString method from MessageInterface as to not confuse message from payload Support for RFC control frames Support message concatenation [BCB] (temporary) WsConnection hard coded to RFC version Handshake checks for \r\n\r\n anywhere, not just at end of string
46 lines
1.2 KiB
PHP
46 lines
1.2 KiB
PHP
<?php
|
|
namespace Ratchet\WebSocket;
|
|
use Ratchet\ConnectionInterface;
|
|
use Ratchet\AbstractConnectionDecorator;
|
|
use Ratchet\WebSocket\Version\VersionInterface;
|
|
use Ratchet\WebSocket\Version\FrameInterface;
|
|
|
|
use Ratchet\WebSocket\Version\RFC6455\Frame;
|
|
|
|
/**
|
|
* {@inheritdoc}
|
|
* @property stdClass $WebSocket
|
|
*/
|
|
class WsConnection extends AbstractConnectionDecorator {
|
|
public function __construct(ConnectionInterface $conn) {
|
|
parent::__construct($conn);
|
|
|
|
$this->WebSocket = new \StdClass;
|
|
}
|
|
|
|
public function send($data) {
|
|
if ($data instanceof FrameInterface) {
|
|
$data = $data->data;
|
|
} elseif (isset($this->WebSocket->version)) {
|
|
// need frame caching
|
|
$data = $this->WebSocket->version->frame($data, false);
|
|
}
|
|
|
|
$this->getConnection()->send($data);
|
|
}
|
|
|
|
/**
|
|
* {@inheritdoc}
|
|
* @todo If code is 1000 send close frame - false is close w/o frame...?
|
|
*/
|
|
public function close($code = 1000) {
|
|
$this->send(Frame::create($code, true, Frame::OP_CLOSE));
|
|
// send close frame with code 1000
|
|
|
|
// ???
|
|
|
|
// profit
|
|
|
|
$this->getConnection()->close(); // temporary
|
|
}
|
|
} |