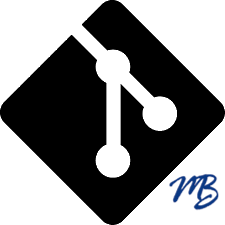
Added the ability to turn UTF-8 encoding checks off to increase performance Separated encoding checks into its own set of classes Encoding checks now use mbstring or iconv depending on availability
31 lines
643 B
PHP
31 lines
643 B
PHP
<?php
|
|
namespace Ratchet\WebSocket\Encoding;
|
|
|
|
class ToggleableValidator implements ValidatorInterface {
|
|
/**
|
|
* Toggle if checkEncoding checks the encoding or not
|
|
* @var bool
|
|
*/
|
|
public $on;
|
|
|
|
/**
|
|
* @var Validator
|
|
*/
|
|
private $validator;
|
|
|
|
public function __construct($on = true) {
|
|
$this->validator = new Validator;
|
|
$this->on = (boolean)$on;
|
|
}
|
|
|
|
/**
|
|
* {@inheritdoc}
|
|
*/
|
|
public function checkEncoding($str, $encoding) {
|
|
if (!(boolean)$this->on) {
|
|
return true;
|
|
}
|
|
|
|
return $this->validator->checkEncoding($str, $encoding);
|
|
}
|
|
} |